210214 Python HackerRank Assignment
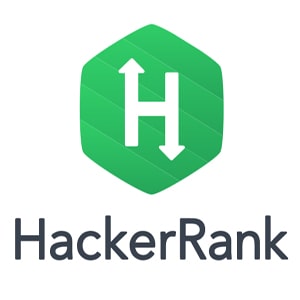
설날 과제로 강사님이 내주신 HackerRank의 과제에 대해서 정리한다.
문제 1
이 문제는 주어진 클래스의 상속관계를 완성하고, 주어진 조건으로 내부에 method를 완성시키면 되는 간단한 문제이다.
1 | class Student(Person): |
210214 Python HackerRank Assignment
설날 과제로 강사님이 내주신 HackerRank의 과제에 대해서 정리한다.
이 문제는 주어진 클래스의 상속관계를 완성하고, 주어진 조건으로 내부에 method를 완성시키면 되는 간단한 문제이다.
1 | class Student(Person): |
210204 Python Regular Expression Assignment
원래 HackerRank 문제의 경우, 별도로 풀이를 정리하지는 않지만, 이번에 정규표현식의 경우, 헷갈리는 부분과 새롭게 공부하게 된 내용이 있어서 별도로 정리를 해본다.
이 문제는 앞 뒤로는 자음만 위치하고, 가운데에는 2개 이상의 모음으로만 구성되어 있는 문자를 정규표현식을 통해 출력하는 문제이다.
Task You are given a string . It consists of alphanumeric characters, spaces and symbols(+, -). Your task is to find all the substrings of that contains or more vowels. Also, these substrings must lie in between consonants and should contain vowels only.
우선 문제를 풀이하기에 앞서 다음 긍정/부정예측(positive/negative lookahead assertion), 긍정 후 읽기에 대한 개념에 대해서 알아보자.
x?=y
: 이 패턴은 직후에 y가 존재하는 문자열 x에 match한다.
1 | foo(?=bar) |
x?!y
: 이 패턴은 직후에 y가 없는 문자열 x에 match한다.
1 | foo(?!bar) |