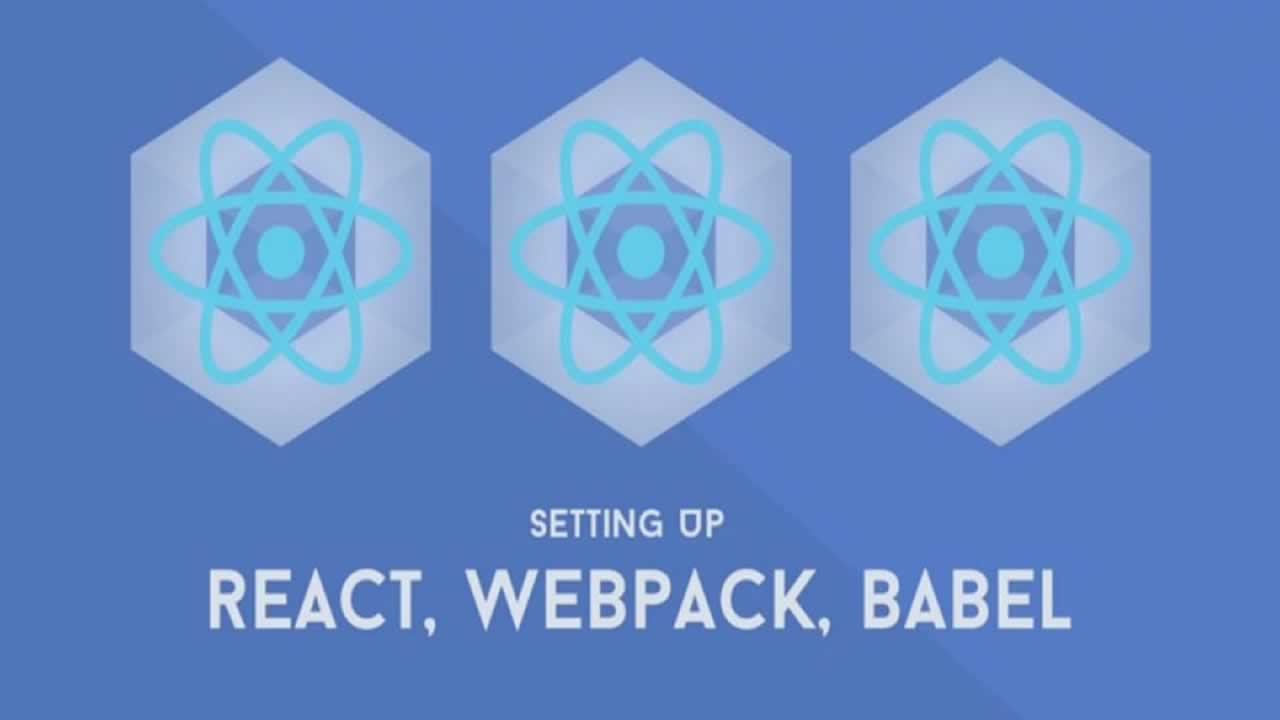
CRA을 사용하지 말고, 나만의 React 프로젝트를 위한 웹팩을 만들자
React프로젝트를 만들때 CRA를 사용해서 손쉽게 React 프로젝트를 생성 할 수 있다. 하지만 이렇게 프로젝트를 생성하다보면, 막상 Webpack을 직접 커스텀해서 해야할때 마냥 어렵게만 느껴지고, 프로젝트에 불필요한 요소들이 자동으로 생성되기 때문에 좋지 않다.
기본적으로 설치해야 될 패키지들은 아래를 참고하자.package.json
1 | "dependencies": { |
webpack5 부터 새롭게 react-refresh를 사용해서 Hot reloading을 구현한다. (아래 webpack 설정파일에 적용)webpack.config.js
1 | const path = require('path'); |
package.json
1 | "scripts": { |