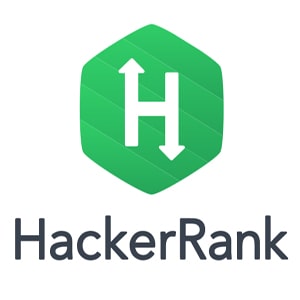
원래 HackerRank 문제의 경우, 별도로 풀이를 정리하지는 않지만, 이번에 정규표현식의 경우, 헷갈리는 부분과 새롭게 공부하게 된 내용이 있어서 별도로 정리를 해본다.
문제 1
이 문제는 앞 뒤로는 자음만 위치하고, 가운데에는 2개 이상의 모음으로만 구성되어 있는 문자를 정규표현식을 통해 출력하는 문제이다.
Task You are given a string . It consists of alphanumeric characters, spaces and symbols(+, -). Your task is to find all the substrings of that contains or more vowels. Also, these substrings must lie in between consonants and should contain vowels only.
우선 문제를 풀이하기에 앞서 다음 긍정/부정예측(positive/negative lookahead assertion), 긍정 후 읽기에 대한 개념에 대해서 알아보자.
긍정적인 예측
x?=y
: 이 패턴은 직후에 y가 존재하는 문자열 x에 match한다.
1 | foo(?=bar) |
부정적인 예측
x?!y
: 이 패턴은 직후에 y가 없는 문자열 x에 match한다.
1 | foo(?!bar) |
긍정 후 읽기
x?<=y
: 이 패턴은 직전에 문자열 x가 있는 문자열 y(x는 포함하지 않음)에 일치한다.
1 | (?<=bar)foo |
부정 후 읽기
x?<!y
: 이 패턴은 직전에 x가 없는 문자열 y에 일치한다.
1 | (?<!bar)foo |
본 코드
1 | import re |
1 | find_object = re.finditer( |
문제 2
이 문제는 입력받은 multiline statements에서 각 문자열에 &&가 있으면 and로, ||가 있으면 or로 변환하는 문제였다.
이 문제를 풀때 처음에는 [\s]로 앞/뒤 공백을 구분하려고 했는데 예상 결과값으로 출력이 안되어, ((?<=[white space]), (?=[white space]))로 다시 정규표현을 작성하여 풀이하였다.
You are given a text of lines. The text contains && and || symbols.
Your task is to modify those symbols to the following:Both && and || should have a space " " on both sides.
&& → and
|| → or
본 코드
1 | import re |
문제 3
이 문제는 입력받은 이메일 주소를 정규표현식(regular expression)을 사용해서 유효성 검사를 하는 문제이다.
이 문제에서는 이전에 배운 meta character를 다양하게 사용해 볼 수 있다.
The first line contains a single integer, , denoting the number of email address.
Each line of the subsequent lines contains a name and an email address as two space-separated values following this format:
name <user@email.com>
본 코드
1 | import re |
문제 4
이문제는 입력받은 전화번호를 정규표현식을 사용해서 유효성 검사를 하는 문제이다.
문제에서 입력받는 전화번호의 조건은 아래와 같다.
condition1) 전화번호는 총 10자리 수로 구성이 된다.
condition2) 앞 자리는 7, 8, 9로 시작한다.
condition3) 나머지 자리수는 숫자(\d)로 구성된다.
본 코드
1 | test_case = int(input()) |